Note
Go to the end to download the full example code.
Source-level RSA using ROI’s#
In this example, we use anatomical labels as Regions Of Interest (ROIs). Rather than using a searchlight, we compute RDMs for each ROI and then compute RSA with a single model RDM.
The dataset will be the MNE-sample dataset: a collection of 288 epochs in which the participant was presented with an auditory beep or visual stimulus to either the left or right ear or visual field.
# sphinx_gallery_thumbnail_number=2
# Import required packages
import mne
import mne_rsa
mne.set_log_level(True) # Be less verbose
mne.viz.set_3d_backend("pyvista")
Using pyvistaqt 3d backend.
We’ll be using the data from the MNE-sample set. To speed up computations in this example, we’re going to use one of the sparse source spaces from the testing set.
sample_root = mne.datasets.sample.data_path(verbose=True)
testing_root = mne.datasets.testing.data_path(verbose=True)
sample_path = sample_root / "MEG" / "sample"
testing_path = testing_root / "MEG" / "sample"
subjects_dir = sample_root / "subjects"
Creating epochs from the continuous (raw) data. We downsample to 100 Hz to speed up the RSA computations later on.
raw = mne.io.read_raw_fif(sample_path / "sample_audvis_filt-0-40_raw.fif")
events = mne.read_events(sample_path / "sample_audvis_filt-0-40_raw-eve.fif")
event_id = {"audio/left": 1, "audio/right": 2, "visual/left": 3, "visual/right": 4}
epochs = mne.Epochs(raw, events, event_id, preload=True)
epochs.resample(100)
Opening raw data file /home/vanvlm1/mne_data/MNE-sample-data/MEG/sample/sample_audvis_filt-0-40_raw.fif...
Read a total of 4 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Average EEG reference (1 x 60) idle
Range : 6450 ... 48149 = 42.956 ... 320.665 secs
Ready.
Not setting metadata
288 matching events found
Setting baseline interval to [-0.19979521315838786, 0.0] s
Applying baseline correction (mode: mean)
Created an SSP operator (subspace dimension = 4)
4 projection items activated
Loading data for 288 events and 106 original time points ...
0 bad epochs dropped
It’s important that the model RDM and the epochs are in the same order, so that each row in the model RDM will correspond to an epoch. The model RDM will be easier to interpret visually if the data is ordered such that all epochs belonging to the same experimental condition are right next to each-other, so patterns jump out. This can be achieved by first splitting the epochs by experimental condition and then concatenating them together again.
epoch_splits = [
epochs[cl] for cl in ["audio/left", "audio/right", "visual/left", "visual/right"]
]
epochs = mne.concatenate_epochs(epoch_splits)
Not setting metadata
288 matching events found
Applying baseline correction (mode: mean)
Created an SSP operator (subspace dimension = 4)
Now that the epochs are in the proper order, we can create a RDM based on the experimental conditions. This type of RDM is referred to as a “sensitivity RDM”. Let’s create a sensitivity RDM that will pick up the left auditory response when RSA-ed against the MEG data. Since we want to capture areas where left beeps generate a large signal, we specify that left beeps should be similar to other left beeps. Since we do not want areas where visual stimuli generate a large signal, we specify that beeps must be different from visual stimuli. Furthermore, since in areas where visual stimuli generate only a small signal, random noise will dominate, we also specify that visual stimuli are different from other visual stimuli. Finally left and right auditory beeps will be somewhat similar.
def sensitivity_metric(event_id_1, event_id_2):
"""Determine similarity between two epochs, given their event ids."""
if event_id_1 == 1 and event_id_2 == 1:
return 0 # Completely similar
if event_id_1 == 2 and event_id_2 == 2:
return 0.5 # Somewhat similar
elif event_id_1 == 1 and event_id_2 == 2:
return 0.5 # Somewhat similar
elif event_id_1 == 2 and event_id_1 == 1:
return 0.5 # Somewhat similar
else:
return 1 # Not similar at all
model_rdm = mne_rsa.compute_rdm(epochs.events[:, 2], metric=sensitivity_metric)
mne_rsa.plot_rdms(model_rdm, title="Model RDM")
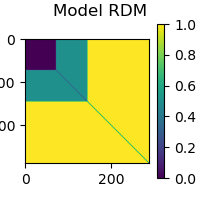
<Figure size 200x200 with 2 Axes>
This example is going to be on source-level, so let’s load the inverse operator and apply it to obtain a cortical surface source estimate for each epoch. To speed up the computation, we going to load an inverse operator from the testing dataset that was created using a sparse source space with not too many vertices.
inv = mne.minimum_norm.read_inverse_operator(
f"{testing_path}/sample_audvis_trunc-meg-eeg-oct-4-meg-inv.fif"
)
epochs_stc = mne.minimum_norm.apply_inverse_epochs(epochs, inv, lambda2=0.1111)
Reading inverse operator decomposition from /home/vanvlm1/mne_data/MNE-testing-data/MEG/sample/sample_audvis_trunc-meg-eeg-oct-4-meg-inv.fif...
Reading inverse operator info...
[done]
Reading inverse operator decomposition...
[done]
305 x 305 full covariance (kind = 1) found.
Read a total of 4 projection items:
PCA-v1 (1 x 102) active
PCA-v2 (1 x 102) active
PCA-v3 (1 x 102) active
Average EEG reference (1 x 60) active
Noise covariance matrix read.
1494 x 1494 diagonal covariance (kind = 2) found.
Source covariance matrix read.
1494 x 1494 diagonal covariance (kind = 6) found.
Orientation priors read.
1494 x 1494 diagonal covariance (kind = 5) found.
Depth priors read.
Did not find the desired covariance matrix (kind = 3)
Reading a source space...
Computing patch statistics...
Patch information added...
[done]
Reading a source space...
Computing patch statistics...
Patch information added...
[done]
2 source spaces read
Read a total of 4 projection items:
PCA-v1 (1 x 102) active
PCA-v2 (1 x 102) active
PCA-v3 (1 x 102) active
Average EEG reference (1 x 60) active
Source spaces transformed to the inverse solution coordinate frame
Preparing the inverse operator for use...
Scaled noise and source covariance from nave = 1 to nave = 1
Created the regularized inverter
Created an SSP operator (subspace dimension = 3)
Created the whitener using a noise covariance matrix with rank 302 (3 small eigenvalues omitted)
Computing noise-normalization factors (dSPM)...
[done]
Picked 305 channels from the data
Computing inverse...
Eigenleads need to be weighted ...
Processing epoch : 1 / 288
combining the current components...
Processing epoch : 2 / 288
combining the current components...
Processing epoch : 3 / 288
combining the current components...
Processing epoch : 4 / 288
combining the current components...
Processing epoch : 5 / 288
combining the current components...
Processing epoch : 6 / 288
combining the current components...
Processing epoch : 7 / 288
combining the current components...
Processing epoch : 8 / 288
combining the current components...
Processing epoch : 9 / 288
combining the current components...
Processing epoch : 10 / 288
combining the current components...
Processing epoch : 11 / 288
combining the current components...
Processing epoch : 12 / 288
combining the current components...
Processing epoch : 13 / 288
combining the current components...
Processing epoch : 14 / 288
combining the current components...
Processing epoch : 15 / 288
combining the current components...
Processing epoch : 16 / 288
combining the current components...
Processing epoch : 17 / 288
combining the current components...
Processing epoch : 18 / 288
combining the current components...
Processing epoch : 19 / 288
combining the current components...
Processing epoch : 20 / 288
combining the current components...
Processing epoch : 21 / 288
combining the current components...
Processing epoch : 22 / 288
combining the current components...
Processing epoch : 23 / 288
combining the current components...
Processing epoch : 24 / 288
combining the current components...
Processing epoch : 25 / 288
combining the current components...
Processing epoch : 26 / 288
combining the current components...
Processing epoch : 27 / 288
combining the current components...
Processing epoch : 28 / 288
combining the current components...
Processing epoch : 29 / 288
combining the current components...
Processing epoch : 30 / 288
combining the current components...
Processing epoch : 31 / 288
combining the current components...
Processing epoch : 32 / 288
combining the current components...
Processing epoch : 33 / 288
combining the current components...
Processing epoch : 34 / 288
combining the current components...
Processing epoch : 35 / 288
combining the current components...
Processing epoch : 36 / 288
combining the current components...
Processing epoch : 37 / 288
combining the current components...
Processing epoch : 38 / 288
combining the current components...
Processing epoch : 39 / 288
combining the current components...
Processing epoch : 40 / 288
combining the current components...
Processing epoch : 41 / 288
combining the current components...
Processing epoch : 42 / 288
combining the current components...
Processing epoch : 43 / 288
combining the current components...
Processing epoch : 44 / 288
combining the current components...
Processing epoch : 45 / 288
combining the current components...
Processing epoch : 46 / 288
combining the current components...
Processing epoch : 47 / 288
combining the current components...
Processing epoch : 48 / 288
combining the current components...
Processing epoch : 49 / 288
combining the current components...
Processing epoch : 50 / 288
combining the current components...
Processing epoch : 51 / 288
combining the current components...
Processing epoch : 52 / 288
combining the current components...
Processing epoch : 53 / 288
combining the current components...
Processing epoch : 54 / 288
combining the current components...
Processing epoch : 55 / 288
combining the current components...
Processing epoch : 56 / 288
combining the current components...
Processing epoch : 57 / 288
combining the current components...
Processing epoch : 58 / 288
combining the current components...
Processing epoch : 59 / 288
combining the current components...
Processing epoch : 60 / 288
combining the current components...
Processing epoch : 61 / 288
combining the current components...
Processing epoch : 62 / 288
combining the current components...
Processing epoch : 63 / 288
combining the current components...
Processing epoch : 64 / 288
combining the current components...
Processing epoch : 65 / 288
combining the current components...
Processing epoch : 66 / 288
combining the current components...
Processing epoch : 67 / 288
combining the current components...
Processing epoch : 68 / 288
combining the current components...
Processing epoch : 69 / 288
combining the current components...
Processing epoch : 70 / 288
combining the current components...
Processing epoch : 71 / 288
combining the current components...
Processing epoch : 72 / 288
combining the current components...
Processing epoch : 73 / 288
combining the current components...
Processing epoch : 74 / 288
combining the current components...
Processing epoch : 75 / 288
combining the current components...
Processing epoch : 76 / 288
combining the current components...
Processing epoch : 77 / 288
combining the current components...
Processing epoch : 78 / 288
combining the current components...
Processing epoch : 79 / 288
combining the current components...
Processing epoch : 80 / 288
combining the current components...
Processing epoch : 81 / 288
combining the current components...
Processing epoch : 82 / 288
combining the current components...
Processing epoch : 83 / 288
combining the current components...
Processing epoch : 84 / 288
combining the current components...
Processing epoch : 85 / 288
combining the current components...
Processing epoch : 86 / 288
combining the current components...
Processing epoch : 87 / 288
combining the current components...
Processing epoch : 88 / 288
combining the current components...
Processing epoch : 89 / 288
combining the current components...
Processing epoch : 90 / 288
combining the current components...
Processing epoch : 91 / 288
combining the current components...
Processing epoch : 92 / 288
combining the current components...
Processing epoch : 93 / 288
combining the current components...
Processing epoch : 94 / 288
combining the current components...
Processing epoch : 95 / 288
combining the current components...
Processing epoch : 96 / 288
combining the current components...
Processing epoch : 97 / 288
combining the current components...
Processing epoch : 98 / 288
combining the current components...
Processing epoch : 99 / 288
combining the current components...
Processing epoch : 100 / 288
combining the current components...
Processing epoch : 101 / 288
combining the current components...
Processing epoch : 102 / 288
combining the current components...
Processing epoch : 103 / 288
combining the current components...
Processing epoch : 104 / 288
combining the current components...
Processing epoch : 105 / 288
combining the current components...
Processing epoch : 106 / 288
combining the current components...
Processing epoch : 107 / 288
combining the current components...
Processing epoch : 108 / 288
combining the current components...
Processing epoch : 109 / 288
combining the current components...
Processing epoch : 110 / 288
combining the current components...
Processing epoch : 111 / 288
combining the current components...
Processing epoch : 112 / 288
combining the current components...
Processing epoch : 113 / 288
combining the current components...
Processing epoch : 114 / 288
combining the current components...
Processing epoch : 115 / 288
combining the current components...
Processing epoch : 116 / 288
combining the current components...
Processing epoch : 117 / 288
combining the current components...
Processing epoch : 118 / 288
combining the current components...
Processing epoch : 119 / 288
combining the current components...
Processing epoch : 120 / 288
combining the current components...
Processing epoch : 121 / 288
combining the current components...
Processing epoch : 122 / 288
combining the current components...
Processing epoch : 123 / 288
combining the current components...
Processing epoch : 124 / 288
combining the current components...
Processing epoch : 125 / 288
combining the current components...
Processing epoch : 126 / 288
combining the current components...
Processing epoch : 127 / 288
combining the current components...
Processing epoch : 128 / 288
combining the current components...
Processing epoch : 129 / 288
combining the current components...
Processing epoch : 130 / 288
combining the current components...
Processing epoch : 131 / 288
combining the current components...
Processing epoch : 132 / 288
combining the current components...
Processing epoch : 133 / 288
combining the current components...
Processing epoch : 134 / 288
combining the current components...
Processing epoch : 135 / 288
combining the current components...
Processing epoch : 136 / 288
combining the current components...
Processing epoch : 137 / 288
combining the current components...
Processing epoch : 138 / 288
combining the current components...
Processing epoch : 139 / 288
combining the current components...
Processing epoch : 140 / 288
combining the current components...
Processing epoch : 141 / 288
combining the current components...
Processing epoch : 142 / 288
combining the current components...
Processing epoch : 143 / 288
combining the current components...
Processing epoch : 144 / 288
combining the current components...
Processing epoch : 145 / 288
combining the current components...
Processing epoch : 146 / 288
combining the current components...
Processing epoch : 147 / 288
combining the current components...
Processing epoch : 148 / 288
combining the current components...
Processing epoch : 149 / 288
combining the current components...
Processing epoch : 150 / 288
combining the current components...
Processing epoch : 151 / 288
combining the current components...
Processing epoch : 152 / 288
combining the current components...
Processing epoch : 153 / 288
combining the current components...
Processing epoch : 154 / 288
combining the current components...
Processing epoch : 155 / 288
combining the current components...
Processing epoch : 156 / 288
combining the current components...
Processing epoch : 157 / 288
combining the current components...
Processing epoch : 158 / 288
combining the current components...
Processing epoch : 159 / 288
combining the current components...
Processing epoch : 160 / 288
combining the current components...
Processing epoch : 161 / 288
combining the current components...
Processing epoch : 162 / 288
combining the current components...
Processing epoch : 163 / 288
combining the current components...
Processing epoch : 164 / 288
combining the current components...
Processing epoch : 165 / 288
combining the current components...
Processing epoch : 166 / 288
combining the current components...
Processing epoch : 167 / 288
combining the current components...
Processing epoch : 168 / 288
combining the current components...
Processing epoch : 169 / 288
combining the current components...
Processing epoch : 170 / 288
combining the current components...
Processing epoch : 171 / 288
combining the current components...
Processing epoch : 172 / 288
combining the current components...
Processing epoch : 173 / 288
combining the current components...
Processing epoch : 174 / 288
combining the current components...
Processing epoch : 175 / 288
combining the current components...
Processing epoch : 176 / 288
combining the current components...
Processing epoch : 177 / 288
combining the current components...
Processing epoch : 178 / 288
combining the current components...
Processing epoch : 179 / 288
combining the current components...
Processing epoch : 180 / 288
combining the current components...
Processing epoch : 181 / 288
combining the current components...
Processing epoch : 182 / 288
combining the current components...
Processing epoch : 183 / 288
combining the current components...
Processing epoch : 184 / 288
combining the current components...
Processing epoch : 185 / 288
combining the current components...
Processing epoch : 186 / 288
combining the current components...
Processing epoch : 187 / 288
combining the current components...
Processing epoch : 188 / 288
combining the current components...
Processing epoch : 189 / 288
combining the current components...
Processing epoch : 190 / 288
combining the current components...
Processing epoch : 191 / 288
combining the current components...
Processing epoch : 192 / 288
combining the current components...
Processing epoch : 193 / 288
combining the current components...
Processing epoch : 194 / 288
combining the current components...
Processing epoch : 195 / 288
combining the current components...
Processing epoch : 196 / 288
combining the current components...
Processing epoch : 197 / 288
combining the current components...
Processing epoch : 198 / 288
combining the current components...
Processing epoch : 199 / 288
combining the current components...
Processing epoch : 200 / 288
combining the current components...
Processing epoch : 201 / 288
combining the current components...
Processing epoch : 202 / 288
combining the current components...
Processing epoch : 203 / 288
combining the current components...
Processing epoch : 204 / 288
combining the current components...
Processing epoch : 205 / 288
combining the current components...
Processing epoch : 206 / 288
combining the current components...
Processing epoch : 207 / 288
combining the current components...
Processing epoch : 208 / 288
combining the current components...
Processing epoch : 209 / 288
combining the current components...
Processing epoch : 210 / 288
combining the current components...
Processing epoch : 211 / 288
combining the current components...
Processing epoch : 212 / 288
combining the current components...
Processing epoch : 213 / 288
combining the current components...
Processing epoch : 214 / 288
combining the current components...
Processing epoch : 215 / 288
combining the current components...
Processing epoch : 216 / 288
combining the current components...
Processing epoch : 217 / 288
combining the current components...
Processing epoch : 218 / 288
combining the current components...
Processing epoch : 219 / 288
combining the current components...
Processing epoch : 220 / 288
combining the current components...
Processing epoch : 221 / 288
combining the current components...
Processing epoch : 222 / 288
combining the current components...
Processing epoch : 223 / 288
combining the current components...
Processing epoch : 224 / 288
combining the current components...
Processing epoch : 225 / 288
combining the current components...
Processing epoch : 226 / 288
combining the current components...
Processing epoch : 227 / 288
combining the current components...
Processing epoch : 228 / 288
combining the current components...
Processing epoch : 229 / 288
combining the current components...
Processing epoch : 230 / 288
combining the current components...
Processing epoch : 231 / 288
combining the current components...
Processing epoch : 232 / 288
combining the current components...
Processing epoch : 233 / 288
combining the current components...
Processing epoch : 234 / 288
combining the current components...
Processing epoch : 235 / 288
combining the current components...
Processing epoch : 236 / 288
combining the current components...
Processing epoch : 237 / 288
combining the current components...
Processing epoch : 238 / 288
combining the current components...
Processing epoch : 239 / 288
combining the current components...
Processing epoch : 240 / 288
combining the current components...
Processing epoch : 241 / 288
combining the current components...
Processing epoch : 242 / 288
combining the current components...
Processing epoch : 243 / 288
combining the current components...
Processing epoch : 244 / 288
combining the current components...
Processing epoch : 245 / 288
combining the current components...
Processing epoch : 246 / 288
combining the current components...
Processing epoch : 247 / 288
combining the current components...
Processing epoch : 248 / 288
combining the current components...
Processing epoch : 249 / 288
combining the current components...
Processing epoch : 250 / 288
combining the current components...
Processing epoch : 251 / 288
combining the current components...
Processing epoch : 252 / 288
combining the current components...
Processing epoch : 253 / 288
combining the current components...
Processing epoch : 254 / 288
combining the current components...
Processing epoch : 255 / 288
combining the current components...
Processing epoch : 256 / 288
combining the current components...
Processing epoch : 257 / 288
combining the current components...
Processing epoch : 258 / 288
combining the current components...
Processing epoch : 259 / 288
combining the current components...
Processing epoch : 260 / 288
combining the current components...
Processing epoch : 261 / 288
combining the current components...
Processing epoch : 262 / 288
combining the current components...
Processing epoch : 263 / 288
combining the current components...
Processing epoch : 264 / 288
combining the current components...
Processing epoch : 265 / 288
combining the current components...
Processing epoch : 266 / 288
combining the current components...
Processing epoch : 267 / 288
combining the current components...
Processing epoch : 268 / 288
combining the current components...
Processing epoch : 269 / 288
combining the current components...
Processing epoch : 270 / 288
combining the current components...
Processing epoch : 271 / 288
combining the current components...
Processing epoch : 272 / 288
combining the current components...
Processing epoch : 273 / 288
combining the current components...
Processing epoch : 274 / 288
combining the current components...
Processing epoch : 275 / 288
combining the current components...
Processing epoch : 276 / 288
combining the current components...
Processing epoch : 277 / 288
combining the current components...
Processing epoch : 278 / 288
combining the current components...
Processing epoch : 279 / 288
combining the current components...
Processing epoch : 280 / 288
combining the current components...
Processing epoch : 281 / 288
combining the current components...
Processing epoch : 282 / 288
combining the current components...
Processing epoch : 283 / 288
combining the current components...
Processing epoch : 284 / 288
combining the current components...
Processing epoch : 285 / 288
combining the current components...
Processing epoch : 286 / 288
combining the current components...
Processing epoch : 287 / 288
combining the current components...
Processing epoch : 288 / 288
combining the current components...
[done]
ROIs need to be defined as mne.Label
objects. Here, we load the APARC parcellation
generated by FreeSurfer and treat each parcel as an ROI.
rois = mne.read_labels_from_annot(
parc="aparc", subject="sample", subjects_dir=subjects_dir
)
Reading labels from parcellation...
read 34 labels from /home/vanvlm1/mne_data/MNE-sample-data/subjects/sample/label/lh.aparc.annot
read 34 labels from /home/vanvlm1/mne_data/MNE-sample-data/subjects/sample/label/rh.aparc.annot
Performing the RSA. To save time, we don’t use a searchlight over time, just over the ROIs. The results are returned not only as a NumPy ndarray, but also as an mne.SourceEstimate object, where each vertex beloning to the same ROI has the same value.
To plot the RSA values on a brain, we can use one of MNE-RSA’s own visualization functions.
brain = mne_rsa.plot_roi_map(
rsa_vals, rois, subject="sample", subjects_dir=subjects_dir
)
brain.show_view("lateral", distance=600)
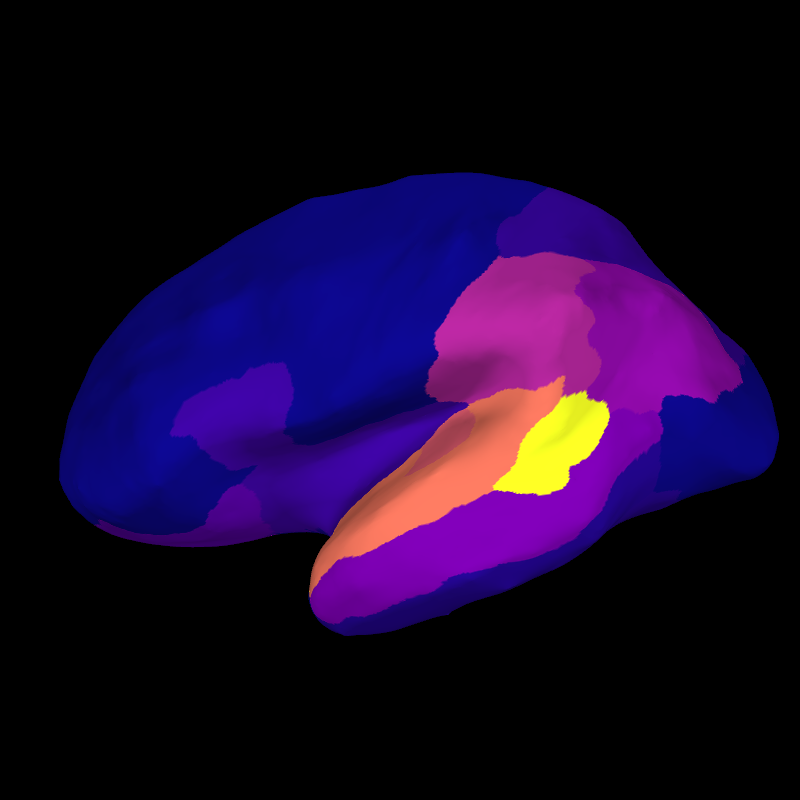
Total running time of the script: (0 minutes 6.444 seconds)